Usage with Shiny
#
Using sketch code in ShinyUse compile_r
to turn a sketch R file into a JavaScript file, copy it into the www
folder of the shiny App, then include the script tag tags$script(src = ...)
in the shiny UI.
#
A full exampleHere is an example of a Shiny App with and without sketch code.
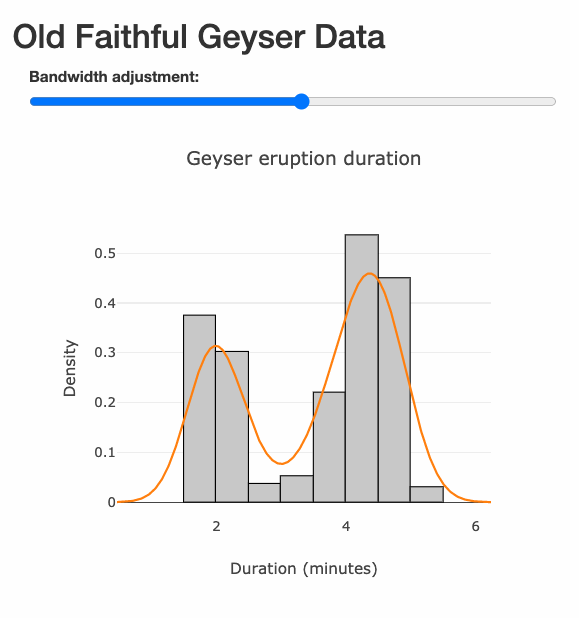
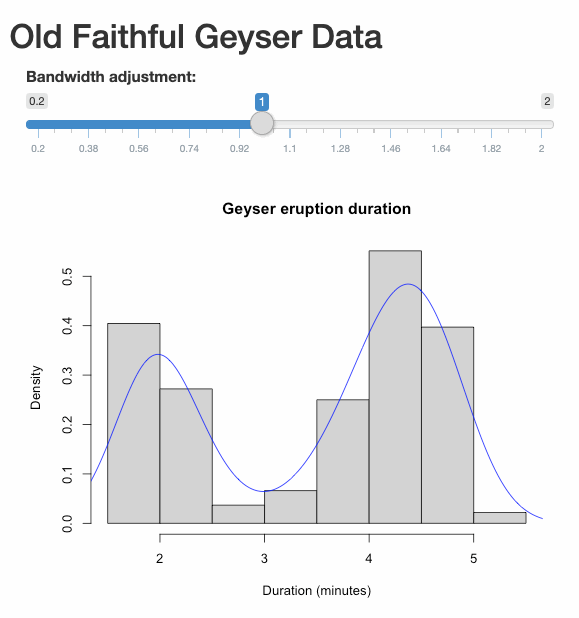
The advantage of using sketch is that it gives you complete control of the JavaScript behaviour, but the disadvantage is of course that more work is needed to achieve such control.
#
Source code#
app.R#
dom.jsdom.js
is provided by the sketch package, and the file path can be found using src("dom")
. Suppose you are at the Shiny App directory, in which there is an www
folder, then dom.js
can be generated by running file.copy(src("dom"), output = "./www/dom.js")
.
#
slider.RGenerate slider.js
using the following command:
#
plot.RGenerate plot.js
using the following command: